Google iCalendar Integration with Aurmur for Direct Booking
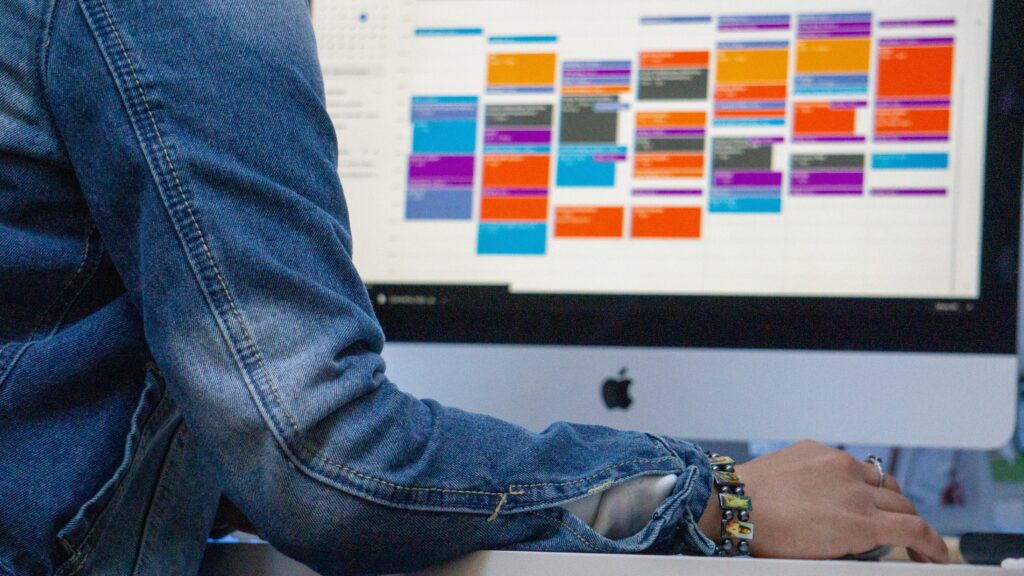
If you’re the owner of a website where guests can book directly with you and want to use Aurmur to generate unique access codes, activate and deactivate them during stays, there’s a simple solution that involves using Google iCalendar. By adding your bookings to a Google iCalendar and providing its URL link when setting up your Aurmur listing, the platform can generate custom codes based on your guests’ phone numbers. These codes will remain valid for the duration of their stay, eliminating the need for you to manually manage access to your property. By streamlining this process, you can offer your guests a seamless and secure experience while also saving time and effort managing your property’s access codes.
Manual way
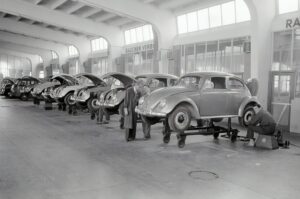
Step 1: Create an event for each booking from your website in your Google’s Reservation iCalendar. Aurmur expects the listing name, guest name in the event title and guest’s phone number in the event description. Below is an example of a booking event for John Doe (phone number 206-555-6666) staying at “Lovely vacation home” between 3/13-3/16.
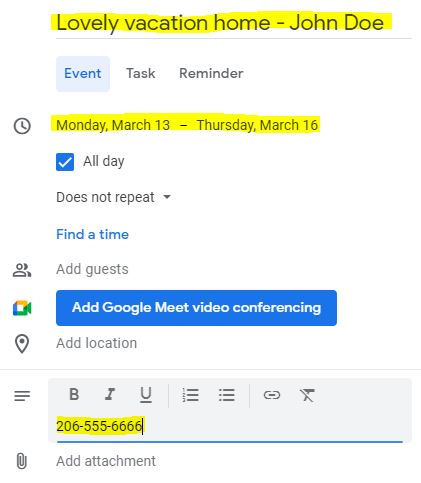
Step 2: Locate the Reservation Calendar URL by clicking the Settings Menu (Gear) icon on the top right and then choose “Settings”.
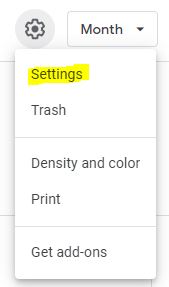
Scroll down to “Settings for my calendars” and click on the Google’s Reservation iCalendar on the left. Scroll down to find the “Integrate calendar” section and locate the public URL of the calendar. This URL will be used by Aurmur.
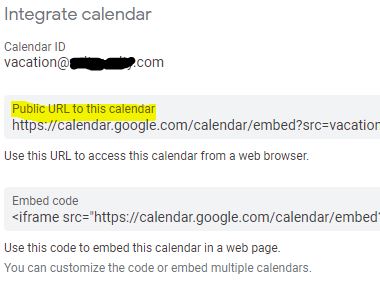
Step 3: Set up the SmartThings hub, account and a smart lock by referring to Onboarding SmartThings blog.
Step 4: Go to LOCKS > LISTINGS to link a listing to the lock. Click “Add Additional Listing”. Give the listing a name under “Listing Name” and click on the corresponding lock under LINKED LOCKS.
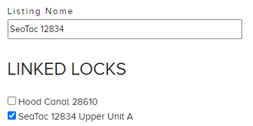
Step 5: Choose No on both Guest Email and Airbnb Message Enabled since they are not available in the booking events.
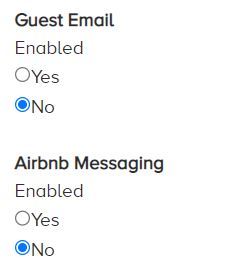
Fortunately, you have the option to configure text message alerts for your guests. You can specify the timing and content of the message that will be sent to them, ensuring that they receive all the necessary information in a timely manner. As an illustration, here’s an example of a text message that could be sent at 11 AM on the day of check-in.

Step 6: Paste the Public URL found in step 2 into one of the three URL boxes under Calendar Integrations. This will enable the integration of your smart lock with your Calendar.
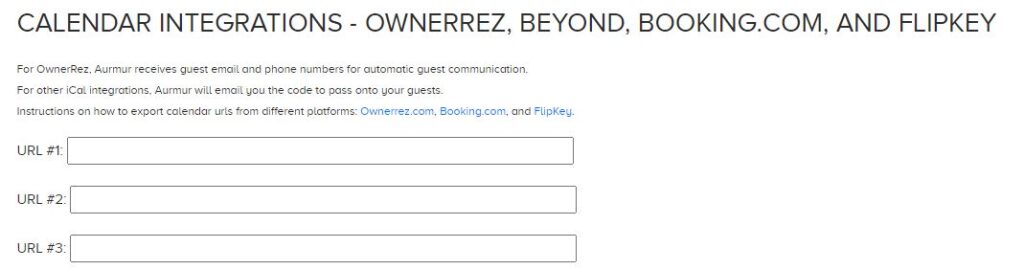
Step 7: In the General Settings section, you can establish door codes that are personalized to your guests’ phone numbers. For additional details on creating custom codes, please consult our the Custom Codes blog.
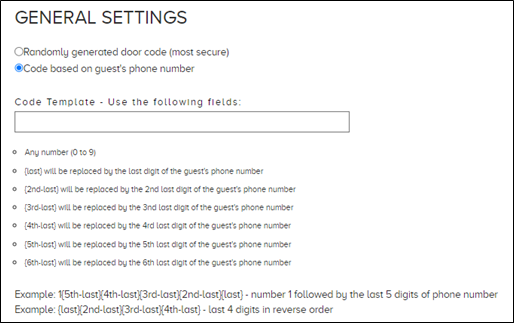
Automatic Way
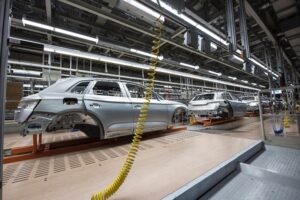
If you’re have developing capability looking to streamline the process of creating booking events on Google iCalendar, here’s a helpful Python code snippet. This code snippet demonstrates how to integrate with Google iCalendar and create booking events automatically, saving you time and effort. With this automation in place, you won’t have to worry about manually creating each booking event and can eliminate step 1 and 2 of the Manual Way section.
from google.oauth2 import service_account
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
from datetime import datetime, timedelta
# Replace with the path to your Google Cloud Platform service account key file
SERVICE_ACCOUNT_FILE = ‘/path/to/service_account_key.json’
# Replace with the calendar ID for the calendar you want to add events to
CALENDAR_ID = ‘your_calendar_id@group.calendar.google.com‘
# Replace with the listing_name, person_name and phone number you want to include in the event
listing_name = ‘Lovely Vacation Home’
guest_name = ‘John Doe’
guest_phone_number = ‘206-555-6666’
start_date_str = ‘2023-03-13’
end_date_str = ‘2023-03-16’
# Set up the credentials object with your Google Cloud Platform service account key file
credentials = service_account.Credentials.from_service_account_file(SERVICE_ACCOUNT_FILE, scopes=[‘https://www.googleapis.com/auth/calendar’])
# Set up the Google Calendar API client
calendar_service = build(‘calendar’, ‘v3’, credentials=credentials)
# Set up the event details
event_start_time = datetime.strptime(start_date_str, ‘%Y-%m-%d’)
event_end_time = datetime.strptime(end_date_str, ‘%Y-%m-%d’).replace(hour=23, minute=59, second=59)
event_summary = f”{listing_name} – {guest_name}”
event_description = f”{guest_phone_number}”
# Create the event
event = {
‘summary’: event_summary,
‘description’: event_description,
‘start’: {
‘dateTime’: event_start_time.isoformat(),
‘timeZone’: ‘UTC’,
},
‘end’: {
‘dateTime’: event_end_time.isoformat(),
‘timeZone’: ‘UTC’,
},
}
# Add the event to the calendar
try:
event = calendar_service.events().insert(calendarId=CALENDAR_ID, body=event).execute()
print(f”Event created: {event.get(‘htmlLink’)}”)
except HttpError as error:
print(f”An error occurred: {error}”)